Signo de colones ansi c
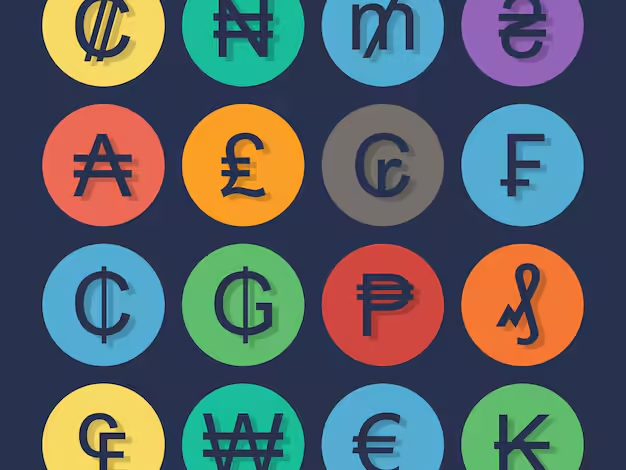
Understanding the Colon Symbol in ANSI C
The colon (:
) symbol in ANSI C is a versatile character that serves several purposes. It is often used in specific programming contexts and syntax structures. Below, we will explore the various uses of the colon in ANSI C programming, including its roles in operators, labels, and conditional statements.
1. Introduction to the Colon Symbol
The colon symbol (:
) is part of the C programming language’s syntax. While it may not be as commonly used as symbols like the semicolon or braces, its functionality is essential for certain programming patterns.
2. Key Uses of the Colon in ANSI C
2.1 Ternary Conditional Operator
The ternary operator is a shorthand for if-else
statements and uses the colon as part of its syntax.
Syntax:
If the condition
evaluates to true
, the expression1
is executed; otherwise, expression2
is executed.
Example:
2.2 Labels for goto
Statements
C supports the goto
statement, which allows jumping to labeled code sections. The colon is used to define labels.
Syntax:
Example:
2.3 Case Labels in switch
Statements
In a switch
statement, the colon separates a case value from its corresponding code block.
Syntax:
Example:
3. Examples of Colon Usage
Example 1: Ternary Operator
Example 2: goto
Labels
4. Common Mistakes and Best Practices
4.1 Common Mistakes
- Misplacing the Colon: Ensure the colon is used in the correct context, such as between expressions in the ternary operator.
- Overusing
goto
: While valid,goto
can make code harder to read and maintain. Use sparingly.
4.2 Best Practices
- Use Readable Syntax: When using ternary operators, avoid overly complex expressions.
- Prefer Structured Programming: Instead of
goto
, use loops or functions to maintain code clarity.
5. Conclusion
The colon symbol in ANSI C plays a critical role in several programming constructs, from conditional expressions to labels and switch statements. While its use is straightforward, understanding its proper application can enhance the clarity and efficiency of your code.
Mastering the colon in ANSI C is a small but significant step toward becoming a proficient C programmer.